序
dubbo
和Spring
、SpringBoot
的集合
环境
Spring (说白了就是两个进程的通信,然后将dubbo交由spring来管理,我们需要做的就是进行配置,然后按照文档硬编码)
<properties> <java.version>1.8</java.version> <spring.version>5.3.4</spring.version> <dubbo.version>2.7.8</dubbo.version> <curator.version>4.2.0</curator.version> <zk.version>3.5.8</zk.version> </properties>
SpringBoot
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.5.4</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.wkk.dubbo</groupId> <artifactId>provider</artifactId> <version>0.0.1-SNAPSHOT</version> <name>provider</name> <description>Demo project for Spring Boot</description> <properties> <java.version>11</java.version> </properties> <dependencies> <!-- https://mvnrepository.com/artifact/org.apache.dubbo/dubbo-spring-boot-starter --> <dependency> <groupId>org.apache.dubbo</groupId> <artifactId>dubbo-spring-boot-starter</artifactId> <version>2.7.13</version> </dependency> <dependency> <groupId>com.wkk.dubbo</groupId> <artifactId>api</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
和Spring的结合(未配置ZK)
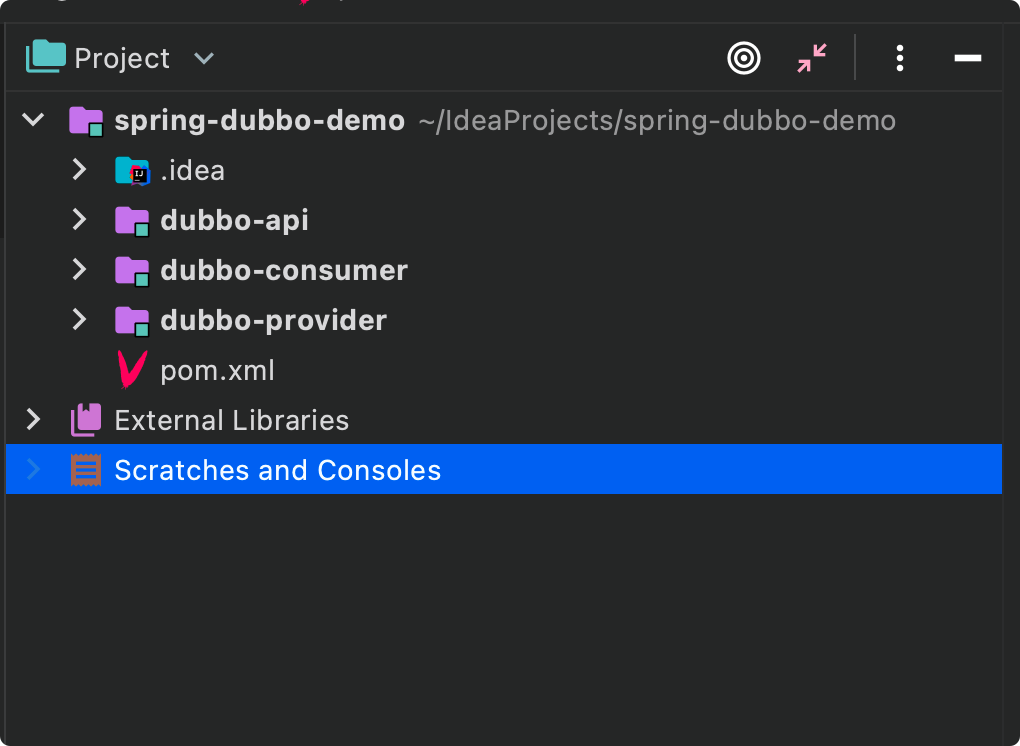
dubbo和spring的结合
pom.xml:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.wkk</groupId>
<artifactId>spring-dubbo-demo</artifactId>
<packaging>pom</packaging>
<version>1.0-SNAPSHOT</version>
<modules>
<module>dubbo-provider</module>
<module>dubbo-consumer</module>
<module>dubbo-api</module>
</modules>
<properties>
<java.version>1.8</java.version>
<spring.version>5.3.4</spring.version>
<dubbo.version>2.7.8</dubbo.version>
<curator.version>4.2.0</curator.version>
<zk.version>3.5.8</zk.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo</artifactId>
<version>${dubbo.version}</version>
</dependency>
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>${zk.version}</version>
</dependency>
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-framework</artifactId>
<version>${curator.version}</version>
</dependency>
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-recipes</artifactId>
<version>${curator.version}</version>
</dependency>
</dependencies>
</project>
API
公共接口,然后
provider
提供对该接口的实现,consume
r对其发起调用
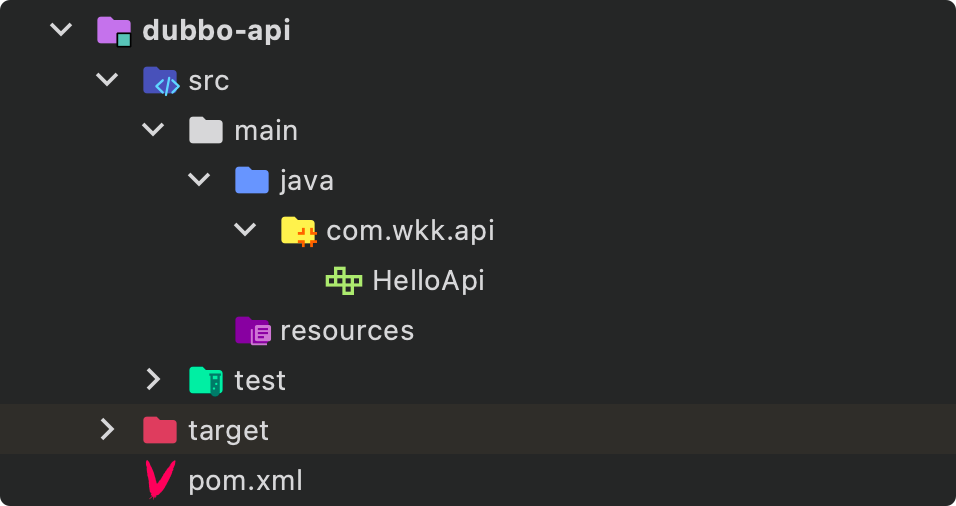
image-20210905233104405
package com.wkk.api;
/**
* @author weikunkun
* @since 2021/9/5
*/
public interface HelloApi {
String hello(String name);
}
PROVIDER
负责对
api
的实现
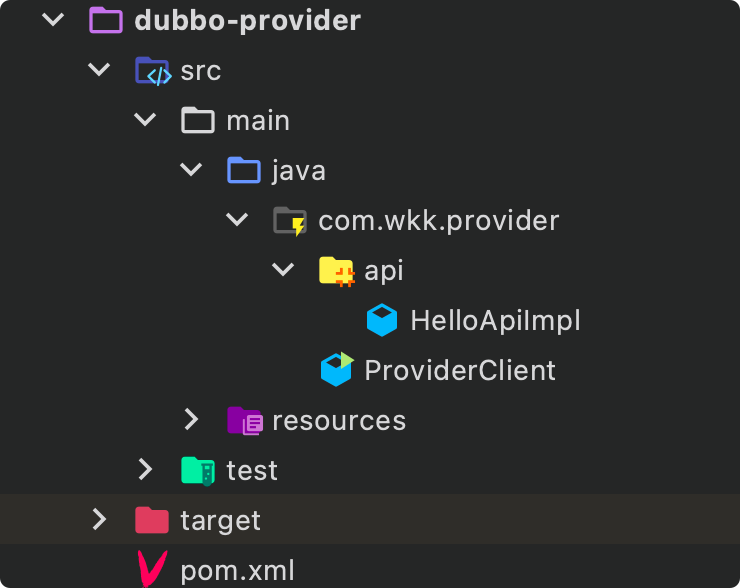
dubbo-provider
配置
<?xml version="1.0" encoding="UTF-8"?>
<!--
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
(the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-->
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://dubbo.apache.org/schema/dubbo"
xmlns="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd">
<dubbo:application name="demo-provider"/>
<dubbo:protocol name="dubbo" port="20880"/>
<!-- 声明对外暴露的接口 -->
<dubbo:service
registry="N/A"
interface="com.wkk.api.HelloApi"
ref="quickStartServiceApi"/>
<!--配置bean实例-->
<bean id="quickStartServiceApi" class="com.wkk.provider.api.HelloApiImpl"/>
</beans>
依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring-dubbo-demo</artifactId>
<groupId>com.wkk</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>dubbo-provider</artifactId>
<dependencies>
<dependency>
<groupId>com.wkk</groupId>
<artifactId>dubbo-api</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
相关实现:
API实现:
package com.wkk.provider.api;
import com.wkk.api.HelloApi;
/**
* @author weikunkun
* @since 2021/9/5
*/
public class HelloApiImpl implements HelloApi {
public String hello(String name) {
return "this is dubbot quick starter, name = " + name;
}
}
启动类:
package com.wkk.provider;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.IOException;
/**
* @author weikunkun
* @since 2021/9/5
*/
public class ProviderClient {
public static void main(String[] args) throws IOException {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("dubbo-provider.xml");
context.start();
System.in.read();
}
}
CONSUMER
负责调用对应的接口,为了方便起见,具体的调用直接在
consumer
中的启动类进行操作
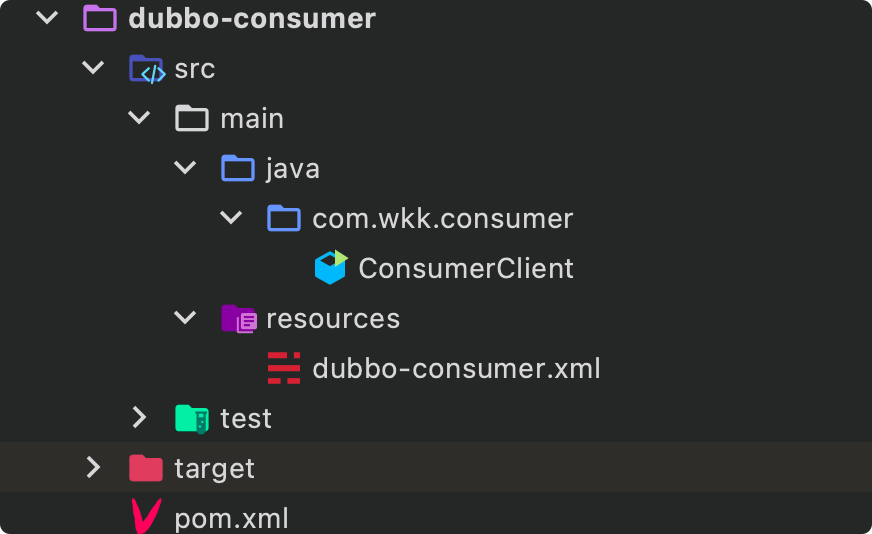
dubbo-consumer
配置
<?xml version="1.0" encoding="UTF-8"?>
<!--
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
(the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-->
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://dubbo.apache.org/schema/dubbo"
xmlns="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd">
<!-- 消费方应用名,用于计算依赖关系,不是匹配条件,不要与提供方一样 -->
<dubbo:application name="consumer-of-helloworld-app" />
<!-- 生成远程服务代理,可以和本地bean一样使用demoService -->
<dubbo:reference id="demoService"
interface="com.wkk.api.HelloApi"
url="dubbo://xxxx:20880"/>
</beans>
依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring-dubbo-demo</artifactId>
<groupId>com.wkk</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>dubbo-consumer</artifactId>
<dependencies>
<dependency>
<groupId>com.wkk</groupId>
<artifactId>dubbo-api</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
相关实现
启动类
package com.wkk.consumer;
import com.wkk.api.HelloApi;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.Scanner;
/**
* @author weikunkun
* @since 2021/9/5
*/
public class ConsumerClient {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("dubbo-consumer.xml");
HelloApi demoService = (HelloApi) context.getBean("demoService");
while (true) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
System.out.println(demoService.hello(str));
}
}
}
最终结果
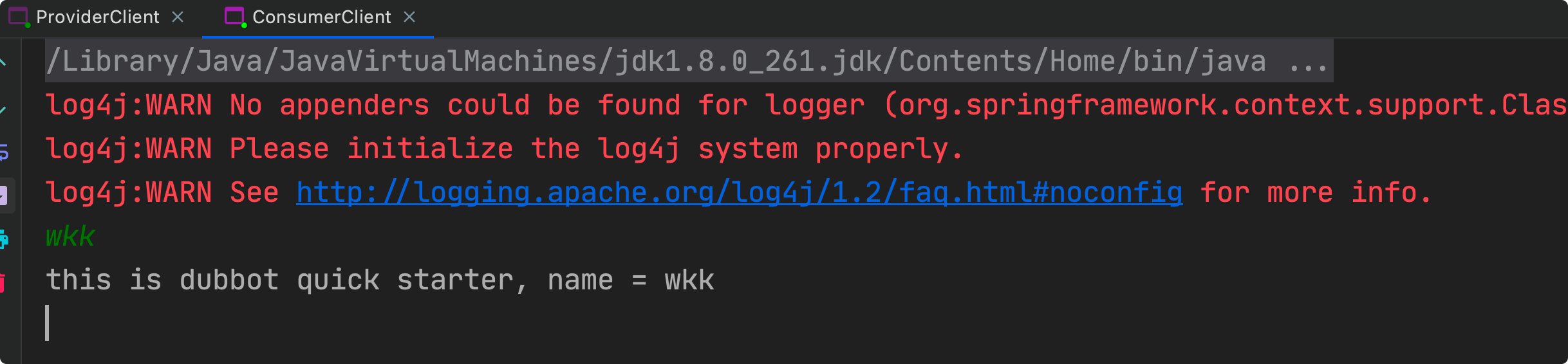
成果展示
和SpringBoot的结合(未配置ZK)
整体和Spring结合一致
API
公共接口,然后
provider
提供对该接口的实现,consume
r对其发起调用
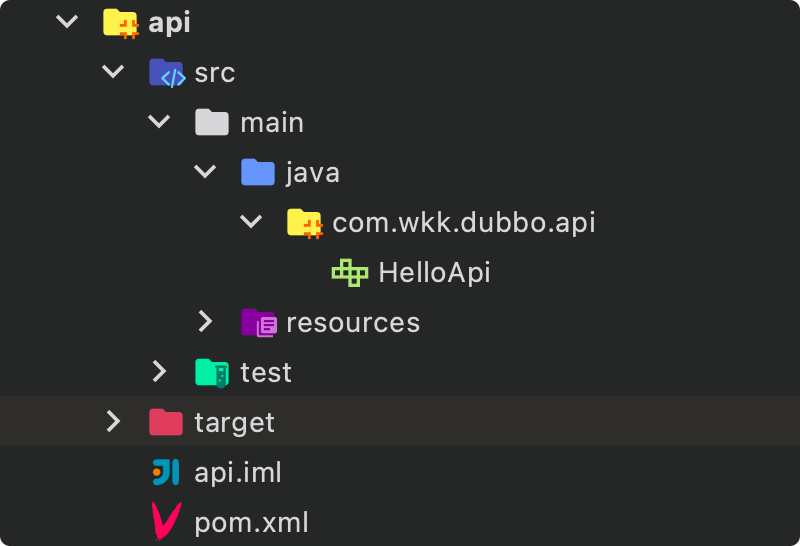
api
接口的设计
package com.wkk.dubbo.api;
/**
* @author weikunkun
* @since 2021/9/6
*/
public interface HelloApi {
String hello(String name);
}
PROVIDER
配置
dubbo:
application:
name: demo-provider
registry:
address: N/A
protocol:
name: dubbo
port: 20880
依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.wkk.dubbo</groupId>
<artifactId>provider</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>provider</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.dubbo/dubbo-spring-boot-starter -->
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>2.7.13</version>
</dependency>
<dependency>
<groupId>com.wkk.dubbo</groupId>
<artifactId>api</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
相关实现
package com.wkk.dubbo.provider.impl;
import com.wkk.dubbo.api.HelloApi;
import org.apache.dubbo.config.annotation.DubboService;
import org.apache.dubbo.rpc.RpcContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
/**
* @author weikunkun
* @since 2021/9/6
*/
@Component
@DubboService(version = "1.0.0")
public class HelloApiImpl implements HelloApi {
private static final Logger logger = LoggerFactory.getLogger(HelloApiImpl.class);
@Override
public String hello(String name) {
try {
Thread.sleep(200); //
logger.info("Hello " + name + ", request from consumer: " + RpcContext.getServerContext().getRemoteAddress());
return "Hello " + name + ", response from provider: " + RpcContext.getServerContext().getLocalAddress();
} catch (InterruptedException e) {
e.printStackTrace();
}
return null;
}
}
CONSUMER
配置
spring:
application:
name: dubbo-consumer
依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.wkk.consumer</groupId>
<artifactId>consumer</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>consumer</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.dubbo/dubbo-spring-boot-starter -->
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>2.7.13</version>
</dependency>
<dependency>
<groupId>com.wkk.dubbo</groupId>
<artifactId>api</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
相关实现
DemoServiceComponent:
用于调用provider中对应的实现方法
package com.wkk.consumer.consumer.comp;
import com.wkk.dubbo.api.HelloApi;
import org.apache.dubbo.config.annotation.DubboReference;
import org.springframework.stereotype.Component;
/**
* @author weikunkun
* @since 2021/9/7
*/
@Component
public class DemoServiceComponent implements HelloApi {
@DubboReference(version = "1.0.0", url = "dubbo://xxxx:20880")
private HelloApi helloApi;
@Override
public String hello(String name) {
return helloApi.hello(name);
}
}
ConsumerApplication:
启动类,通过Scanner做了交互
package com.wkk.consumer.consumer;
import com.wkk.dubbo.api.HelloApi;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
import java.util.Scanner;
@SpringBootApplication
public class ConsumerApplication {
public static void main(String[] args) {
ConfigurableApplicationContext context = SpringApplication.run(ConsumerApplication.class, args);
HelloApi demoService = (HelloApi) context.getBean("demoServiceComponent");
Scanner sc = new Scanner(System.in);
while (true) {
String s = sc.nextLine();
System.out.println(demoService.hello(s));
}
}
}
最终结果
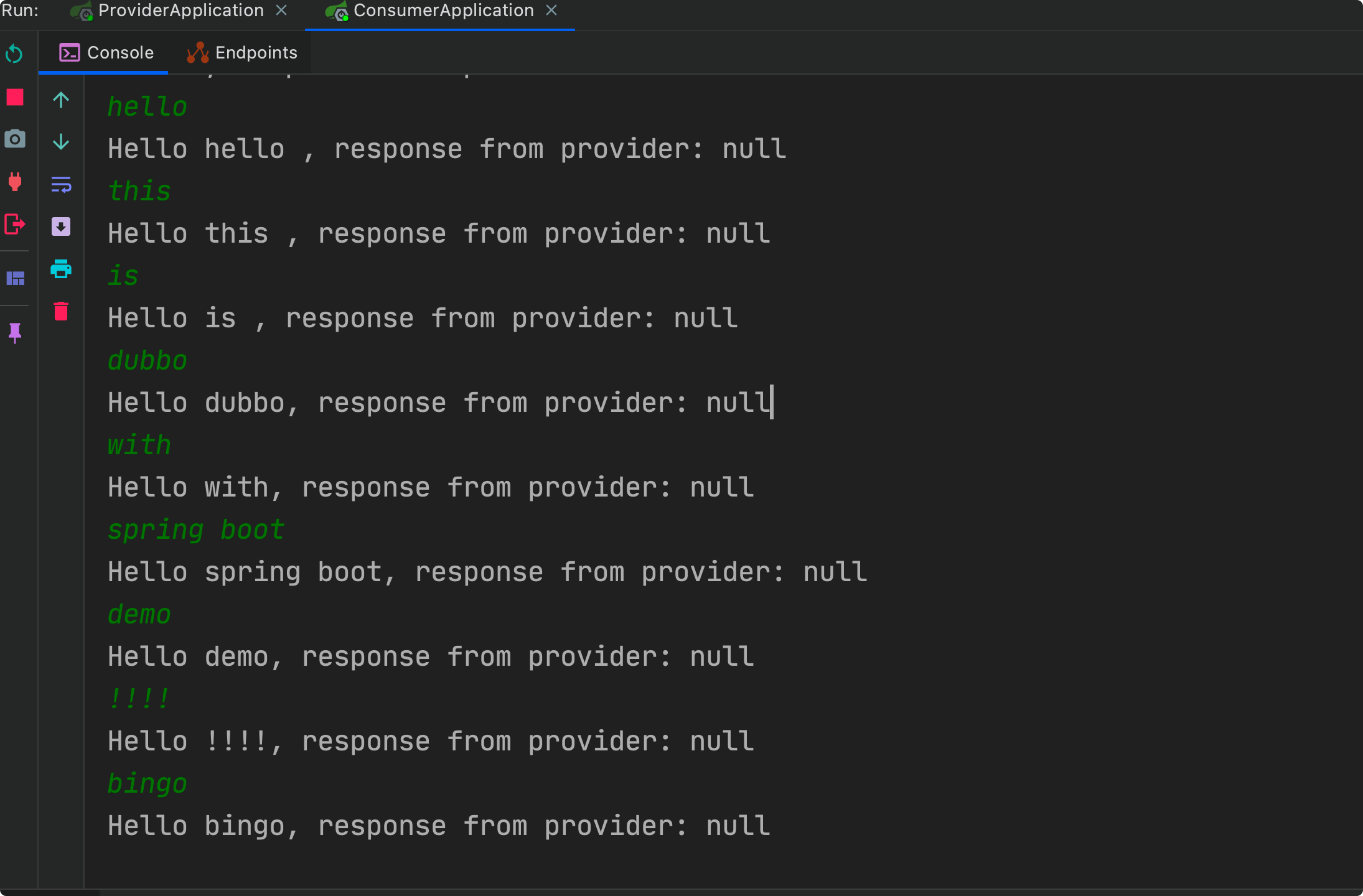
成果展示